~
Auto update NO-IP DNS with python
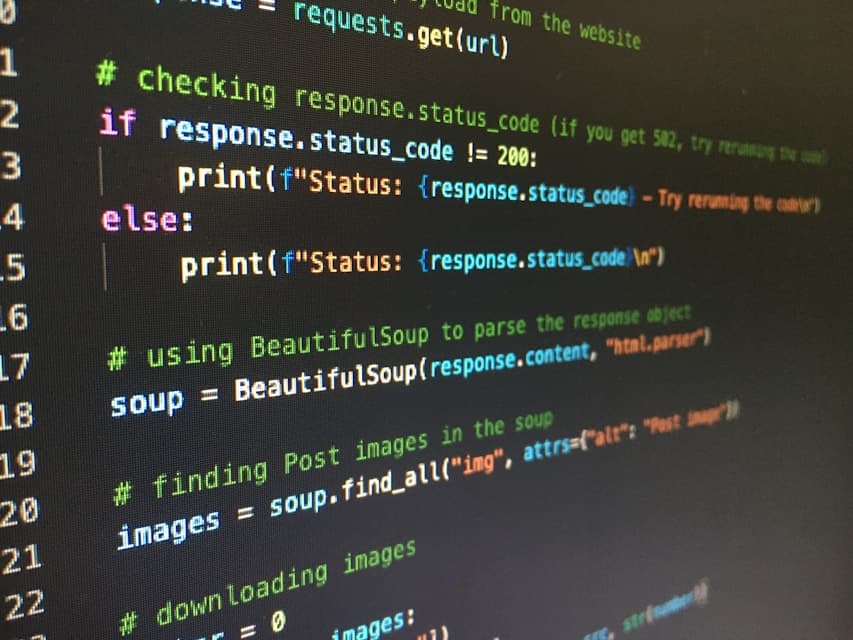
If you're using NO-IP DNS service and want to automatically update your DNS records with your local IP address, you can achieve this by using a Python script and scheduling it to run periodically with crontab. In this blog post, we'll walk through the process of setting it up.
Prerequisites
Before we get started, make sure you have the following:
- Python installed on your machine
- A NO-IP account with a registered hostname
- Username and password for your NO-IP account
- Basic knowledge of Python
Step 1: Setting up the Python script
-
Create a new Python file, for example, noip_update.py, and open it in a text editor or an integrated development environment (IDE).
-
Copy the following code into the Python file:
from urllib.request import urlopen
import requests
import re
import datetime
def get_my_ip():
resp_url = str(urlopen('http://checkip.dyndns.org/').read())
ip = re.findall(r"\d+\.\d+\.\d+\.\d+", resp_url)[0]
return ip
def update_ip_on_no_ip(my_ip: str, u_name: str, passwd: str, host_name: str):
url = f"https://{u_name}:{passwd}@dynupdate.no-ip.com/nic/update?hostname={host_name}&myip={my_ip}"
payload = {}
headers = {}
try:
response = requests.request("GET", url, headers=headers, data=payload)
except Exception as e:
print(e)
print(f"Error occurred while doing update: {e}\n")
return False
return response.text
u_name = "username"
passwd = "password"
host_name = "mytest.example.com"
ip_update = get_my_ip()
update_ip_on_no_ip(
my_ip=ip_update,
u_name=u_name,
passwd=passwd,
host_name=host_name
)
Note: Replace the username, password, and hostname with your own. You can find your hostname in the NO-IP dashboard.
- Save the file in place where you can easily find it later. (For example, "/home/user/noip_update.py")
Step 2: Testing the Python script
-
Open a terminal or command prompt.
-
Navigate to the directory where you saved the Python file.
-
Run the following command to execute the Python script:
python3 noip_update.py
- The script will retrieve your local IP address and update it on your NO-IP account. If successful, it will display the response from the update request. If not, it will display an error message. So check the response to make sure it was successful.
Step 3: Scheduling the script with crontab
Now that we have a working Python script, let's schedule it to run automatically at regular intervals using crontab.
-
Open a terminal or command prompt.
-
Run the following command to open the crontab configuration file:
crontab -e
Note: If prompted, choose an editor to open the file.
- Add the following line at the end of the file to run the Python script every 5 minutes:
*/5 * * * * python /home/user/noip_update.py
-
Make sure to replace /home/user/noip_update.py with the actual path to your Python file.
-
Save the file and exit the editor.
Crontab will automatically pick up the changes, and your Python script will now run every 5 minutes to update your NO-IP DNS with your local IP.
Conclusion
And that's it! You've successfully set up auto-updating of your NO-IP DNS using a Python script and crontab. Your DNS records will now stay up to date with your local IP without any manual intervention.
Feel free to customize the intervals or add additional error handling in the Python script to suit your needs. Remember to secure your Python script by setting appropriate file permissions and protecting your credentials.
I hope this blog post helps you automate the process of updating your NO-IP DNS with your local IP address. If you have any questions or run into any issues, feel free to ask for help. Happy updating!
References
Story Time
I have many projects running on my home server, and I wanted to access them from outside my home network. I didn't want to pay for a static IP address, so I decided to use a dynamic DNS service.
I chose NO-IP because it's free and easy to set up. But I didn't want to manually update my DNS records every time my IP address changed. So I wrote a Python script to do it for me.
I scheduled the script to run every 5 minutes using crontab, and now my DNS records stay up to date with my local IP address without any manual intervention.
Special Thanks
Photo by Artturi Jalli on Unsplash
Comments
Feel free to share your thoughts, feedback, or questions about upgrading IP address with NO-IP DNS using Python in the comments section below. Let's engage in meaningful discussions and explore the endless possibilities of automating your DNS updates!
Please Note: Sometimes, the comments might not show up. If that happens, just refresh the page, and they should appear.