~
Setting Up ChatGPT with Python: A Simple Guide
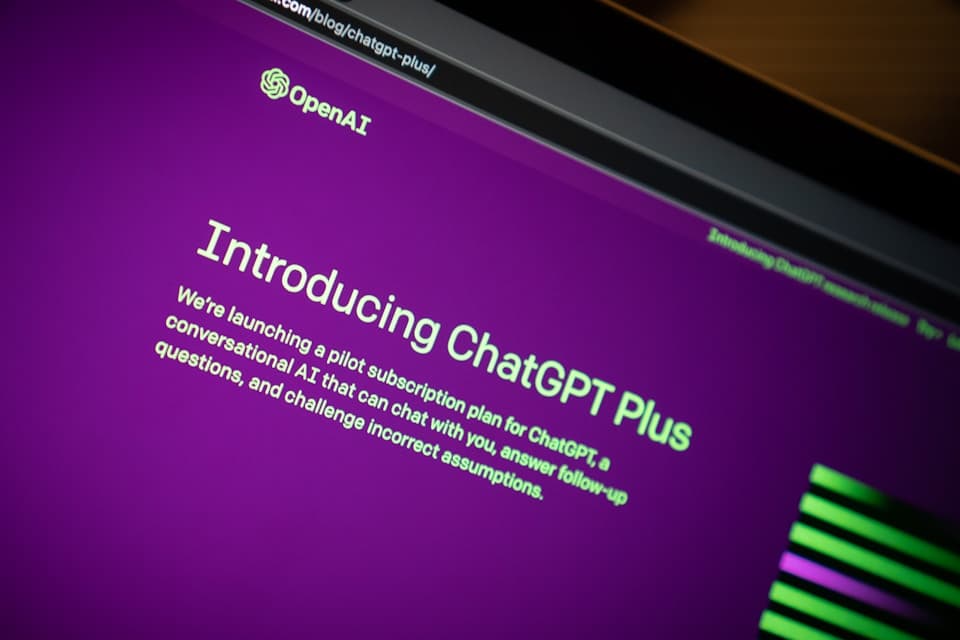
ChatGPT, powered by the revolutionary GPT-3.5 architecture developed by OpenAI, allows developers to create engaging and interactive chatbot experiences. In this blog post, we will explore how to set up ChatGPT using Python, providing you with a simple step-by-step guide to get started.
Prerequisites
To follow along with this guide, you should have a basic understanding of Python programming and have Python installed on your machine. Additionally, make sure to have the OpenAI Python library installed. You can install it by running the following command:
pip3 install openai
Step 1: Obtain OpenAI API Key
To access the power of ChatGPT, you'll need an OpenAI API key. Visit the OpenAI website and sign up for an account if you haven't already. Once you have an account, obtain your API key from the OpenAI dashboard.
Step 2: Import Libraries and Authenticate
Open your Python editor or Jupyter notebook and import the required libraries. In this case, we need the openai
library. Additionally, authenticate your OpenAI API key by using the following code snippet:
import openai
# Authenticate using your API key
openai.api_key = 'YOUR_API_KEY'
Replace
'YOUR_API_KEY'
with the actual API key you obtained in Step 1.
Step 3: Create a ChatGPT Session
To interact with ChatGPT, we need to create a session. This session will store the conversation history and allow us to send messages back and forth. Here's how you can create a session:
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
]
)
session_id = response['id']
Note that we use the
"gpt-3.5-turbo"
model, which is the most advanced model at the time of writing. Feel free to experiment with other models depending on your requirements.
Step 4: Interact with ChatGPT
Now that we have our session ready, we can start interacting with ChatGPT. Here's an example of sending a user message and receiving a response:
def send_message(session_id, message):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": message},
],
session_id=session_id
)
return response['choices'][0]['message']['content']
# Example conversation loop
while True:
user_input = input("User: ")
response = send_message(session_id, user_input)
print("ChatGPT: " + response)
Step 5: Customize and Improve
ChatGPT's performance can be enhanced by customizing the instructions and tweaking the conversation format. Experiment with different conversation formats and instructions to achieve the desired results. For example, you can provide initial messages or instructions to guide ChatGPT's behavior and make it more helpful and context-aware.
Conclusion
In this blog post, we explored a simple guide to set up ChatGPT using Python. We covered the key steps, including obtaining an API key, authenticating, creating a session, and interacting with ChatGPT. With this knowledge, you can now harness the power of ChatGPT to create engaging and interactive chatbot experiences. Remember to experiment and customize the instructions and conversation format to optimize your chatbot's performance. Happy coding!
References
Story Time
I use ChatGPT to help me with my projects. I have a lot of projects going on at the same time. I am using this in the production also where partial automation is required. This was a excellent choice for us.
So, this was the reason why I started using ChatGPT and its python library.
Special Thanks
Photo by Jonathan Kemper on Unsplash
Comments
Feel free to share your thoughts, feedback, or questions about setting up ChatGPT with Python in the comments section below. Let's engage in meaningful discussions and explore the endless possibilities of AI-powered chatbots!
Please Note: Sometimes, the comments might not show up. If that happens, just refresh the page, and they should appear.