~
7 Essential Python Libraries Every Developer Should Know
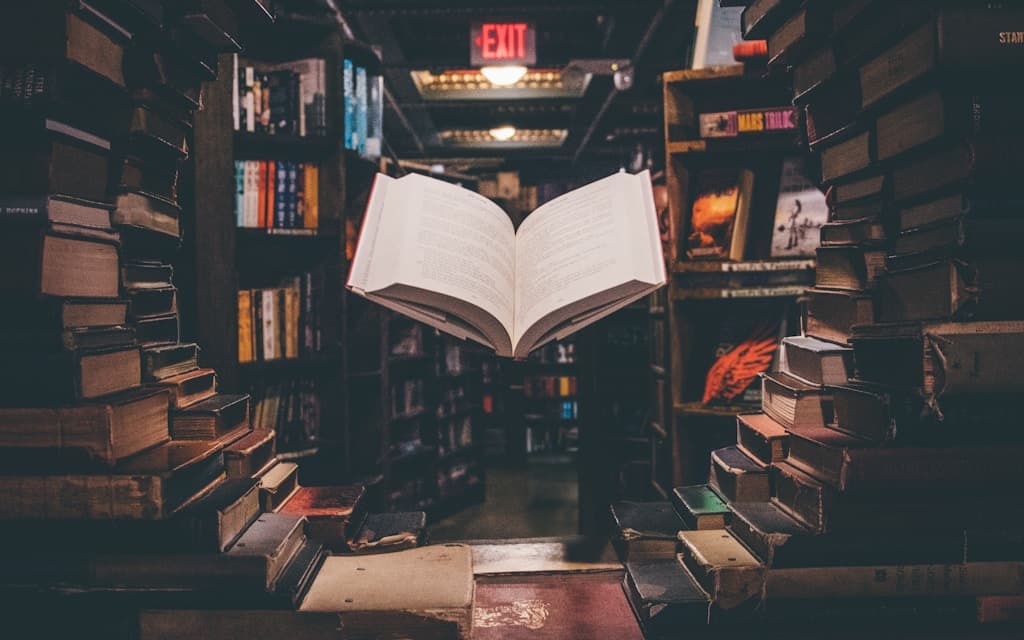
Python is a versatile and powerful programming language that offers a vast ecosystem of libraries and modules. These libraries provide additional functionality and simplify complex tasks, allowing developers to write more efficient and effective code. In this article, we will explore seven essential Python libraries that every developer should know. From logging to operating system interactions, these libraries will enhance your programming experience and make your code more robust.
1. Logging
Learn how to log the output of your programs to easily debug and understand what's happening in your code.
Logging is a critical aspect of software development. It allows you to record information about the execution of your program, making it easier to debug issues and understand the flow of your code. The Python logging
library provides a flexible and configurable way to log messages in your application.
Here's a simple example of how to use the logging
library:
import logging
# Configure the logger
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
# Log messages
logging.debug('Debug message')
logging.info('Info message')
logging.warning('Warning message')
logging.error('Error message')
logging.critical('Critical message')
In this example, we import the logging
module and configure the logger to display messages with a specified format and log level. We then use different log levels (debug
, info
, warning
, error
, critical
) to log messages at different levels of severity.
2. Regular Expressions (re)
Discover the power of working with regular expressions to find patterns and extract data from strings.
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. The Python re
module provides support for working with regular expressions, allowing you to search, match, and manipulate strings based on specific patterns.
Let's see a simple example of how to use the re
library:
import re
# Define a pattern
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b'
# Test string
text = "Contact us at info@example.com or support@example.org"
# Search for email addresses
matches = re.findall(pattern, text)
# Print the matches
for match in matches:
print(match)
In this example, we define a regular expression pattern to match email addresses. We then use the findall
function from the re
module to search for all occurrences of the pattern in the given text. The resulting matches are printed to the console.
3. Pandas
Dive into data analysis and manipulation with Pandas, the go-to library for working with datasets of any size.
Pandas is a powerful library for data analysis and manipulation. It provides data structures and functions to efficiently work with structured data, such as CSV files or database tables. With Pandas, you can perform various operations like filtering, grouping, and merging datasets, making it an essential tool for data scientists and analysts.
Here's a simple example showcasing some of Pandas' capabilities:
import pandas as pd
# Create a DataFrame
data = {'Name': ['John', 'Emma', 'Peter'],
'Age': [25, 28, 31],
'Country': ['USA', 'Canada', 'UK']}
df = pd.DataFrame(data)
# Print the DataFrame
print(df)
# Filter the data
filtered_df = df[df['Age'] > 25]
# Print the filtered DataFrame
print(filtered_df)
In this example, we create a DataFrame using a dictionary. We then print the DataFrame and apply a filter to select rows where the 'Age' column is greater than 25. The resulting filtered DataFrame is printed to the console.
4. NumPy
Explore NumPy's capabilities in handling arrays and matrices, essential for scientific computing and numerical operations.
NumPy is a fundamental library for scientific computing and numerical operations in Python. It provides powerful tools for working with arrays, matrices, and mathematical functions, making it indispensable for tasks involving numerical data.
Let's see a basic example of using NumPy:
import numpy as np
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Perform operations on the array
mean = np.mean(arr)
sum = np.sum(arr)
# Print the results
print("Mean:", mean)
print("Sum:", sum)
In this example, we import NumPy as np
and create an array. We then use NumPy functions to calculate the mean and sum of the array. Finally, we print the results to the console.
5. JSON
Unleash the potential of working with JSON data, a lightweight and widely-used data-interchange format.
JSON (JavaScript Object Notation) is a popular data-interchange format used for transmitting and storing structured data. Python's built-in json
module allows you to work with JSON data effortlessly, converting JSON strings to Python objects and vice versa.
Here's a simple example of using the json
library:
import json
# JSON data
data = '{"name": "John", "age": 30, "city": "New York"}'
# Parse JSON into a Python object
obj = json.loads(data)
# Access the data
print("Name:", obj['name'])
print("Age:", obj['age'])
print("City:", obj['city'])
# Convert Python object to JSON
json_data = json.dumps(obj)
print("JSON data:", json_data)
In this example, we start with a JSON string and use json.loads()
to parse it into a Python object. We can then access the data using key-value pairs. Additionally, we demonstrate converting a Python object back into a JSON string using json.dumps()
.
6. Typing
Harness the benefits of type hints with the typing library, improving code readability and catching bugs early.
Python 3.5 introduced type hints as a way to add type information to function and variable annotations. The typing
module, available in later Python versions, enhances code readability and enables static type checking using tools like mypy
.
Here's a simple example demonstrating the usage of type hints:
from typing import List
def square_numbers(numbers: List[int]) -> List[int]:
return [num ** 2 for num in numbers]
# Usage
result = square_numbers([1, 2, 3, 4, 5])
print(result)
In this example, we use the List
type hint from the typing
module to indicate that the numbers
parameter of the square_numbers
function should be a list of integers. Similarly, the return type hint indicates that the function will return a list of integers. This helps improve code readability and allows tools like mypy
to detect potential type-related issues.
7. OS
Get access to powerful operating system functionalities, such as file system interaction, path manipulation, and executing system commands.
The os
module provides a simple and consistent interface for interacting with the underlying operating system. It offers various functions for file and directory operations, path manipulation, environment variables, and executing system commands.
Here's a simple example showcasing the os
module:
import os
# Get the current working directory
cwd = os
.getcwd()
print("Current directory:", cwd)
# List files in a directory
files = os.listdir('.')
print("Files in current directory:", files)
# Execute a system command
os.system('ls -l')
In this example, we use the os.getcwd()
function to retrieve the current working directory and os.listdir()
to list the files in the current directory. Finally, we execute the ls -l
command using os.system()
.
Conclusion
These seven Python libraries, namely logging, regular expressions (re), Pandas, NumPy, JSON, typing, and OS, offer essential functionalities that can significantly enhance your programming experience. Whether you need to log messages, work with data, manipulate arrays, handle JSON, add type hints, or interact with the operating system, these libraries provide the necessary tools to simplify your code and increase productivity. By familiarizing yourself with these libraries and their capabilities, you'll become a more proficient Python developer ready to tackle a wide range of programming challenges.
References
Story Time
I was using the 7 listed libraries in my Python projects a lot, these libraries are very useful and powerful. I checked all my projects and found that I used these libraries in almost all of them, then I thought it would be a good idea to share these libraries with you.
So, I made this article to share these libraries with you, I hope you find it useful.
Special Thanks
Photo by Jaredd Craig on Unsplash
Comments
Feel free to share your thoughts, feedback, or questions about the essential Python libraries mentioned in this article. Let's engage in meaningful discussions and explore the endless possibilities of Python programming!
Please Note: Sometimes, the comments might not show up. If that happens, just refresh the page, and they should appear.